大模型调用
智谱AI
智普AI 我们这边可以使用GLM-4-Flash,免费的但是会限制速率
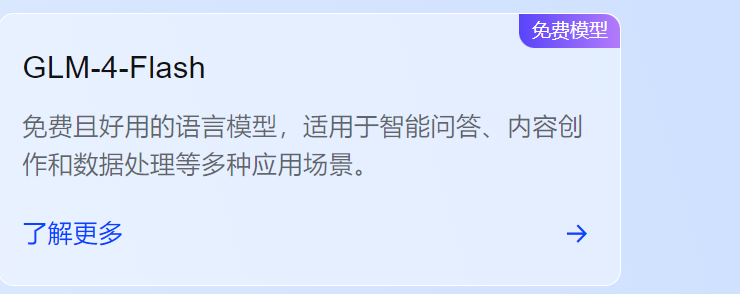

需要先申请Key,https://www.bigmodel.cn/usercenter/proj-mgmt/apikeys
初次注册会送免费的token
1. Token Utils
package icu.flycode.sdk.utils;
import com.auth0.jwt.JWT;
import com.auth0.jwt.algorithms.Algorithm;
import com.google.common.cache.Cache;
import com.google.common.cache.CacheBuilder;
import java.nio.charset.StandardCharsets;
import java.util.Calendar;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.TimeUnit;
public class BearerTokenUtils {
// 过期时间;默认30分钟
private static final long EXPIRE_MILLIS = 30 * 60 * 1000L;
// 缓存服务
public static Cache<String, String> cache = CacheBuilder.newBuilder()
.expireAfterWrite(EXPIRE_MILLIS - (60 * 1000L), TimeUnit.MILLISECONDS)
.build();
public static String getToken(String apiKeySecret) {
String[] split = apiKeySecret.split("\\.");
return getToken(split[0], split[1]);
}
/**
* 对 ApiKey 进行签名
*
* @param apiKey 登录创建 ApiKey <a href="https://open.bigmodel.cn/usercenter/apikeys">apikeys</a>
* @param apiSecret apiKey的后半部分 828902ec516c45307619708d3e780ae1.w5eKiLvhnLP8MtIf 取 w5eKiLvhnLP8MtIf 使用
* @return Token
*/
public static String getToken(String apiKey, String apiSecret) {
// 缓存Token
String token = cache.getIfPresent(apiKey);
if (null != token) {
return token;
}
// 创建Token
Algorithm algorithm = Algorithm.HMAC256(apiSecret.getBytes(StandardCharsets.UTF_8));
Map<String, Object> payload = new HashMap<>();
payload.put("api_key", apiKey);
payload.put("exp", System.currentTimeMillis() + EXPIRE_MILLIS);
payload.put("timestamp", Calendar.getInstance().getTimeInMillis());
Map<String, Object> headerClaims = new HashMap<>();
headerClaims.put("alg", "HS256");
headerClaims.put("sign_type", "SIGN");
token = JWT.create().withPayload(payload).withHeader(headerClaims).sign(algorithm);
cache.put(apiKey, token);
return token;
}
}
2. 生成Token
public class ApiTest {
public static void main(String[] args) {
String apiKey = "xxxx";
String token = BearerTokenUtils.getToken(apiKey);
System.out.println(token);
}
}
这个生成的token会在调用的时候需要
3. 编写curl脚本
curl -X POST \
-H "Authorization: Bearer token" \
-H "Content-Type: application/json" \
-H "User-Agent: Mozilla/4.0 (compatible; MSIE 5.0; Windows NT; DigExt)" \
-d '{
"model":"glm-4",
"stream": "true",
"messages": [
{
"role": "user",
"content": "1+1"
}
]
}' \
https://open.bigmodel.cn/api/paas/v4/chat/completions
将上面的curl脚本使用ApiFox或者其他方式请求数据
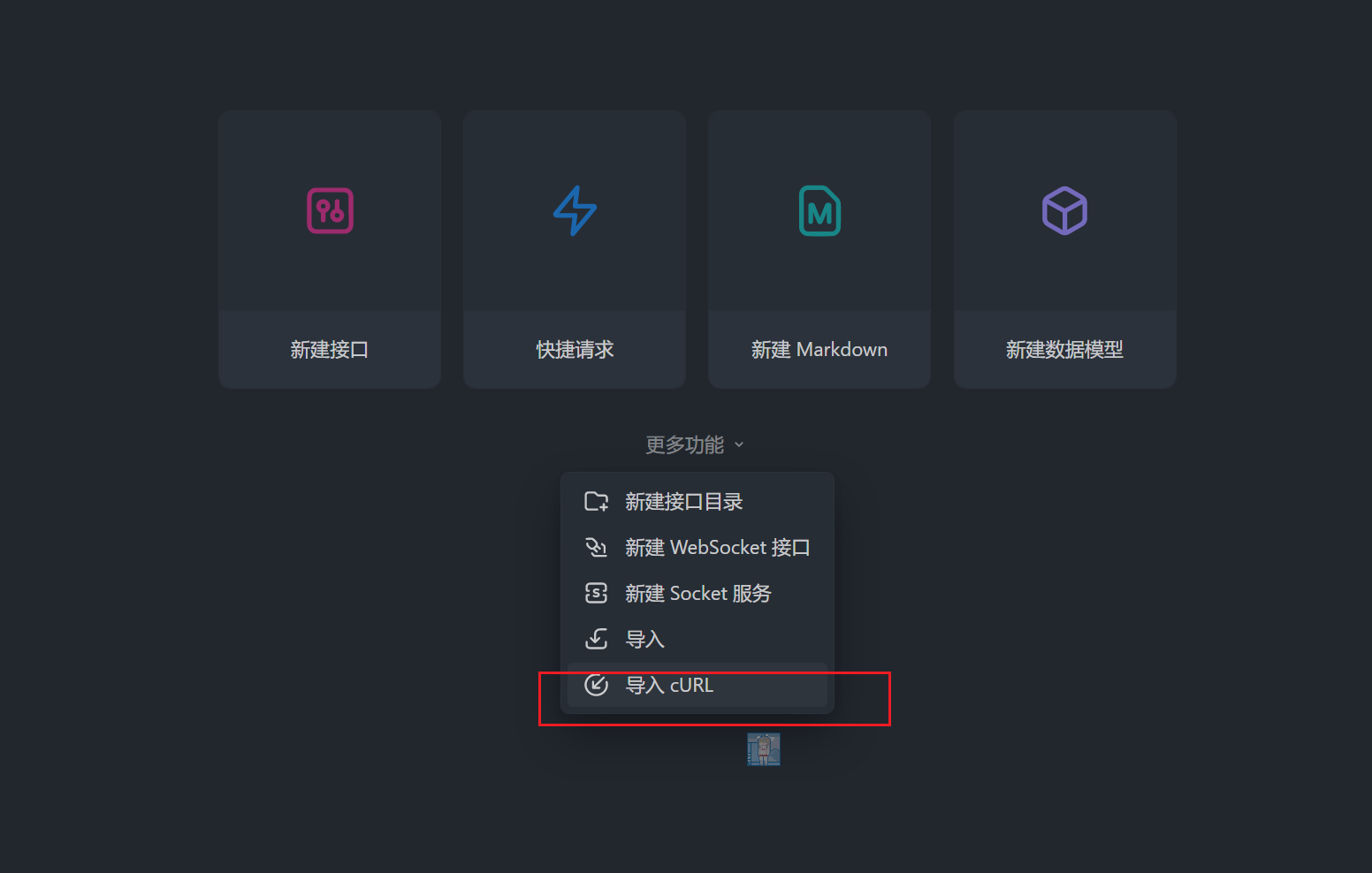
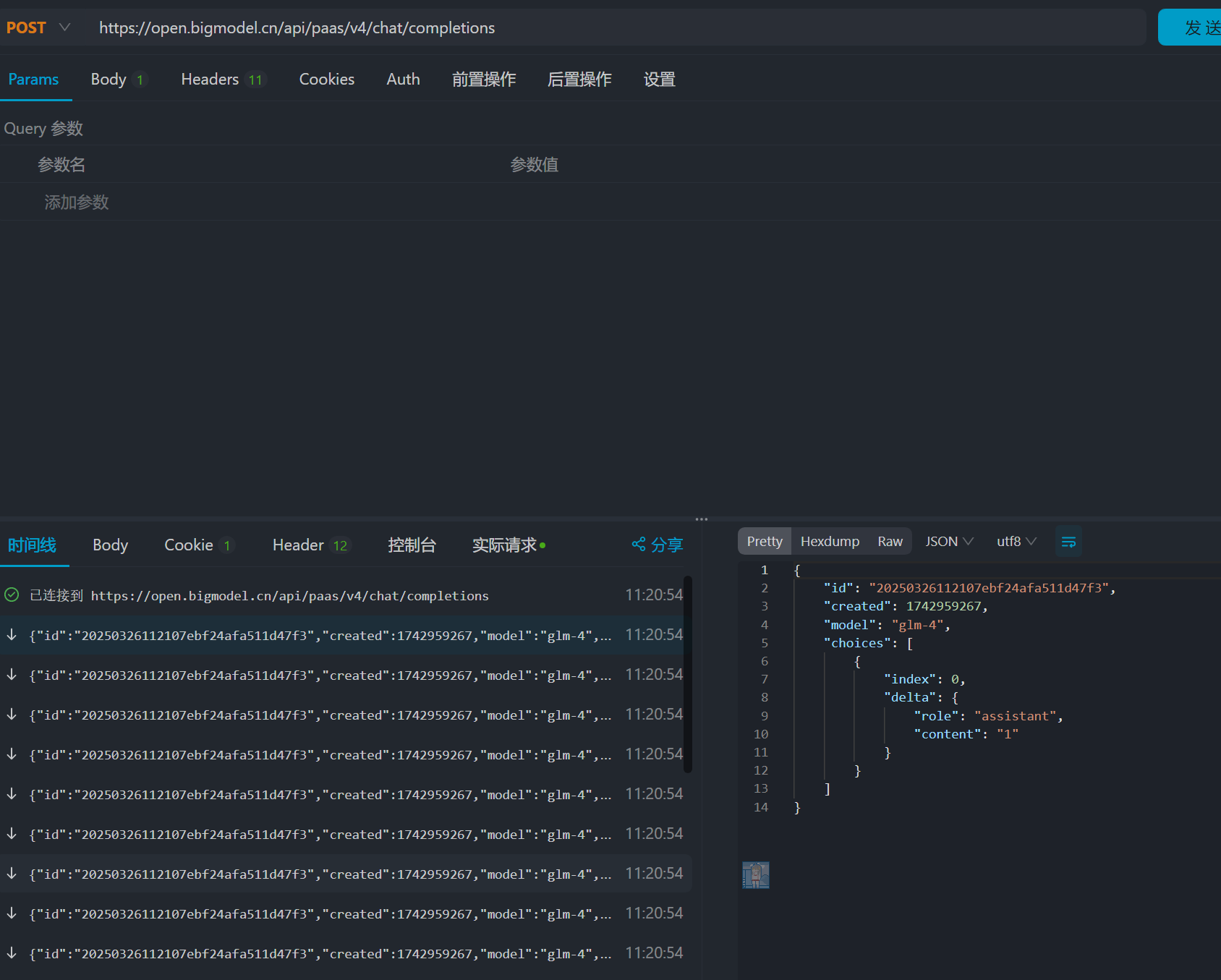
4. Java测试代码
主要步骤如下:
- 构造http发送请求,格式如上面的curl
- 解析返回的数据
@Test
public void test_http() throws IOException {
String apiKey = "xxxxxx";
String token = BearerTokenUtils.getToken(apiKey);
URL url = new URL(" https://open.bigmodel.cn/api/paas/v4/chat/completions");
HttpsURLConnection httpsURLConnection = (HttpsURLConnection) url.openConnection();
httpsURLConnection.setRequestMethod("POST");
httpsURLConnection.setRequestProperty("Authorization", "Bearer " + token);
httpsURLConnection.setRequestProperty("Content-Type", "application/json");
httpsURLConnection.setRequestProperty("User-Agent", "Mozilla/4.0 (compatible; MSIE 5.0; Windows NT; DigExt)");
httpsURLConnection.setDoOutput(true);
String code = "print('Hello, World!')";
String jsonInputString = "{"
+ "\"model\":\"glm-4-flash\","
+ "\"messages\": ["
+ " {"
+ " \"role\": \"user\","
+ " \"content\": \"你是一个高级编程架构师,精通各类场景方案、架构设计和编程语言,根据用户提交的代码分析给出解决方案。代码为: " + code + "\""
+ " }"
+ "]"
+ "}";
try (OutputStream os = httpsURLConnection.getOutputStream()) {
byte[] input = jsonInputString.getBytes("utf-8");
os.write(input, 0, input.length);
}
int responseCode = httpsURLConnection.getResponseCode();
System.out.println(responseCode);
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(httpsURLConnection.getInputStream()));
String line;
StringBuilder content = new StringBuilder();
while ((line = bufferedReader.readLine()) != null) {
content.append(line);
}
bufferedReader.close();
httpsURLConnection.disconnect();
System.out.println(content);
}

我们只需要将里面的内容转换为实体对象,即可获取content内容。
5. 编写模型发送响应实体类
package icu.flycode.sdk.domain.models;
public enum Model {
@Deprecated
CHATGLM_6B_SSE("chatGLM_6b_SSE", "ChatGLM-6B 测试模型"),
@Deprecated
CHATGLM_LITE("chatglm_lite", "轻量版模型,适用对推理速度和成本敏感的场景"),
@Deprecated
CHATGLM_LITE_32K("chatglm_lite_32k", "标准版模型,适用兼顾效果和成本的场景"),
@Deprecated
CHATGLM_STD("chatglm_std", "适用于对知识量、推理能力、创造力要求较高的场景"),
@Deprecated
CHATGLM_PRO("chatglm_pro", "适用于对知识量、推理能力、创造力要求较高的场景"),
/** 智谱AI 23年06月发布 */
CHATGLM_TURBO("chatglm_turbo", "适用于对知识量、推理能力、创造力要求较高的场景"),
/** 智谱AI 24年01月发布 */
GLM_3_5_TURBO("glm-3-turbo","适用于对知识量、推理能力、创造力要求较高的场景"),
GLM_4("glm-4","适用于复杂的对话交互和深度内容创作设计的场景"),
GLM_4V("glm-4v","根据输入的自然语言指令和图像信息完成任务,推荐使用 SSE 或同步调用方式请求接口"),
GLM_4_FLASH("glm-4-flash","适用简单任务,速度最快,价格最实惠的版本,具有128k上下文"),
COGVIEW_3("cogview-3","根据用户的文字描述生成图像,使用同步调用方式请求接口"),
;
private final String code;
private final String info;
Model(String code, String info) {
this.code = code;
this.info = info;
}
public String getCode() {
return code;
}
public String getInfo() {
return info;
}
}
发送请求设置角色
import java.util.List;
public class ChatCompletionRequest {
private String model = Model.GLM_4_FLASH.getCode();
private List<Prompt> messages;
public static class Prompt {
private String role;
private String content;
public Prompt() {
}
public Prompt(String role, String content) {
this.role = role;
this.content = content;
}
public String getRole() {
return role;
}
public void setRole(String role) {
this.role = role;
}
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
}
public String getModel() {
return model;
}
public void setModel(String model) {
this.model = model;
}
public List<Prompt> getMessages() {
return messages;
}
public void setMessages(List<Prompt> messages) {
this.messages = messages;
}
}
获取响应
package icu.flycode.sdk.domain.models;
import java.util.List;
public class ChatCompletionSyncResponse {
private List<Choice> choices;
public static class Choice {
private Message message;
public Message getMessage() {
return message;
}
public void setMessage(Message message) {
this.message = message;
}
}
public static class Message {
private String role;
private String content;
public String getRole() {
return role;
}
public void setRole(String role) {
this.role = role;
}
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
}
public List<Choice> getChoices() {
return choices;
}
public void setChoices(List<Choice> choices) {
this.choices = choices;
}
}
6. 修改测试代码
public static void main(String[] args) throws Exception {
System.out.println("测试执行");
// 代码评审
// 1. 读取Git Diff更改记录
ProcessBuilder processBuilder = new ProcessBuilder("git", "diff", "HEAD~1", "HEAD");
processBuilder.directory(new File("."));
Process process = processBuilder.start();
// 读取输出流
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(process.getInputStream()));
String line;
StringBuilder diffStr = new StringBuilder();
while ((line = bufferedReader.readLine()) != null) {
diffStr.append(line);
}
// 2. 获取退出码
int exitCode = process.waitFor();
System.out.println("Exited with code: " + exitCode);
// 3. 返回读取数据
System.out.println("diff code: " + diffStr.toString());
// 4. 调用OpenAI API进行代码评审
String codedReview = codeReview(diffStr.toString());
System.out.println("Code review: " + codedReview);
}
private static String codeReview(String diffCode) throws Exception {
String apiKey = "046183b32b904844949bd062b1ab223c.MEgwXNBvYeLMvd51";
String token = BearerTokenUtils.getToken(apiKey);
HttpsURLConnection httpsURLConnection = getHttpsURLConnection(token);
ChatCompletionRequest chatCompletionRequest = new ChatCompletionRequest();
chatCompletionRequest.setModel(Model.GLM_4_FLASH.getCode());
chatCompletionRequest.setMessages(new ArrayList<ChatCompletionRequest.Prompt>(){
{
add(new ChatCompletionRequest.Prompt("user","你是一个高级编程架构师,精通各类场景方案、架构设计和编程语言请,请您根据git diff记录,对代码做出评审。代码为: "));
add(new ChatCompletionRequest.Prompt("user",diffCode));
}
});
try (OutputStream os = httpsURLConnection.getOutputStream()) {
byte[] input = JSON.toJSONString(chatCompletionRequest).getBytes(StandardCharsets.UTF_8);
os.write(input, 0, input.length);
}
int responseCode = httpsURLConnection.getResponseCode();
System.out.println(responseCode);
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(httpsURLConnection.getInputStream()));
String line;
StringBuilder content = new StringBuilder();
while ((line = bufferedReader.readLine()) != null) {
content.append(line);
}
bufferedReader.close();
httpsURLConnection.disconnect();
System.out.println(content);
ChatCompletionSyncResponse chatCompletionSyncResponse = JSON.parseObject(content.toString(), ChatCompletionSyncResponse.class);
String returnContent = chatCompletionSyncResponse.getChoices().get(0).getMessage().getContent();
return returnContent;
}
private static HttpsURLConnection getHttpsURLConnection(String token) throws IOException {
URL url = new URL("https://open.bigmodel.cn/api/paas/v4/chat/completions");
HttpsURLConnection httpsURLConnection = (HttpsURLConnection) url.openConnection();
httpsURLConnection.setRequestMethod("POST");
httpsURLConnection.setRequestProperty("Authorization", "Bearer " + token);
httpsURLConnection.setRequestProperty("Content-Type", "application/json");
httpsURLConnection.setRequestProperty("User-Agent", "Mozilla/4.0 (compatible; MSIE 5.0; Windows NT; DigExt)");
httpsURLConnection.setDoOutput(true);
return httpsURLConnection;
}
贡献者
flycodeu
版权所有
版权归属:flycodeu