微信模板消息测试
测试公众号地址
http://mp.weixin.qq.com/debug/cgi-bin/sandboxinfo?action=showinfo&t=sandbox/index

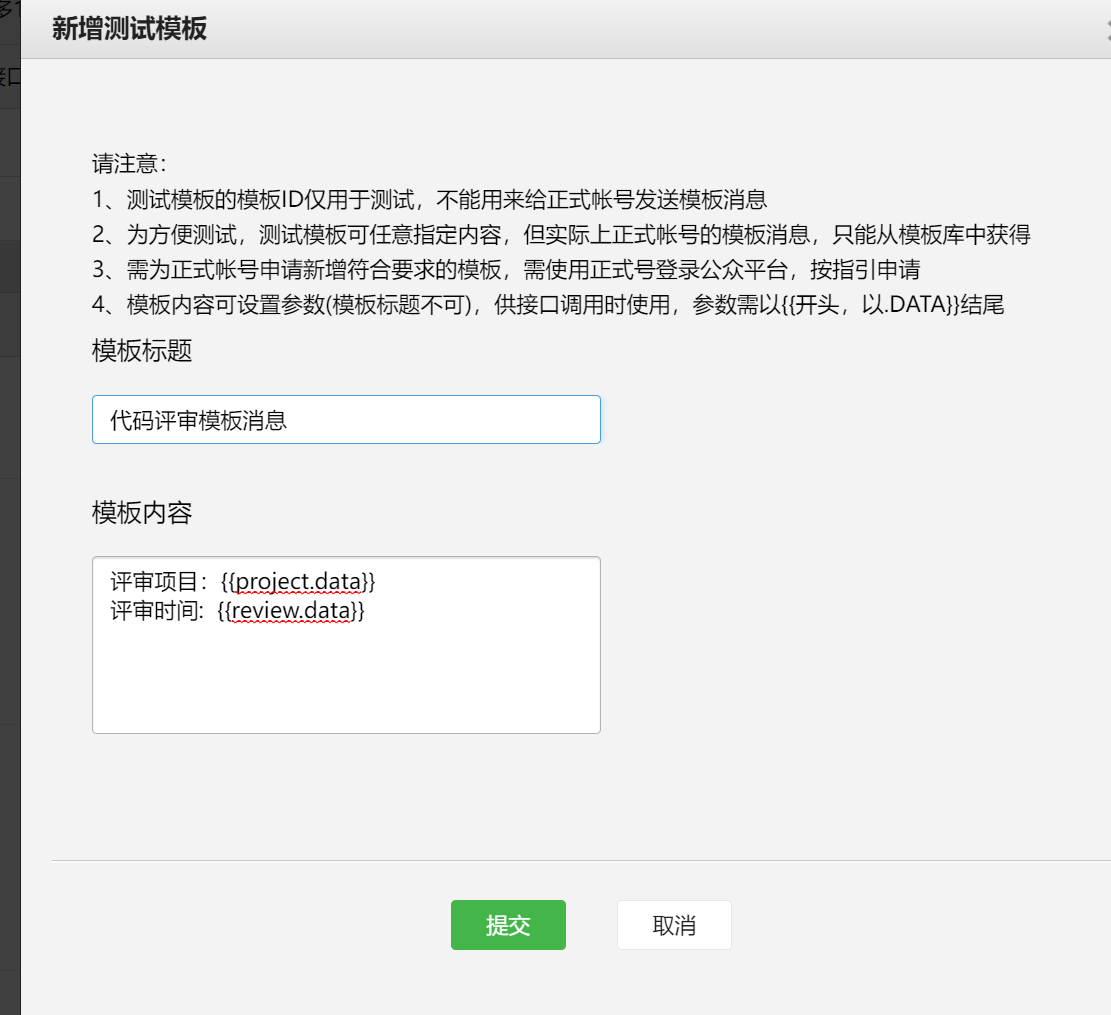
调用地址:https://mp.weixin.qq.com/debug/cgi-bin/readtmpl?t=tmplmsg/faq_tmpl
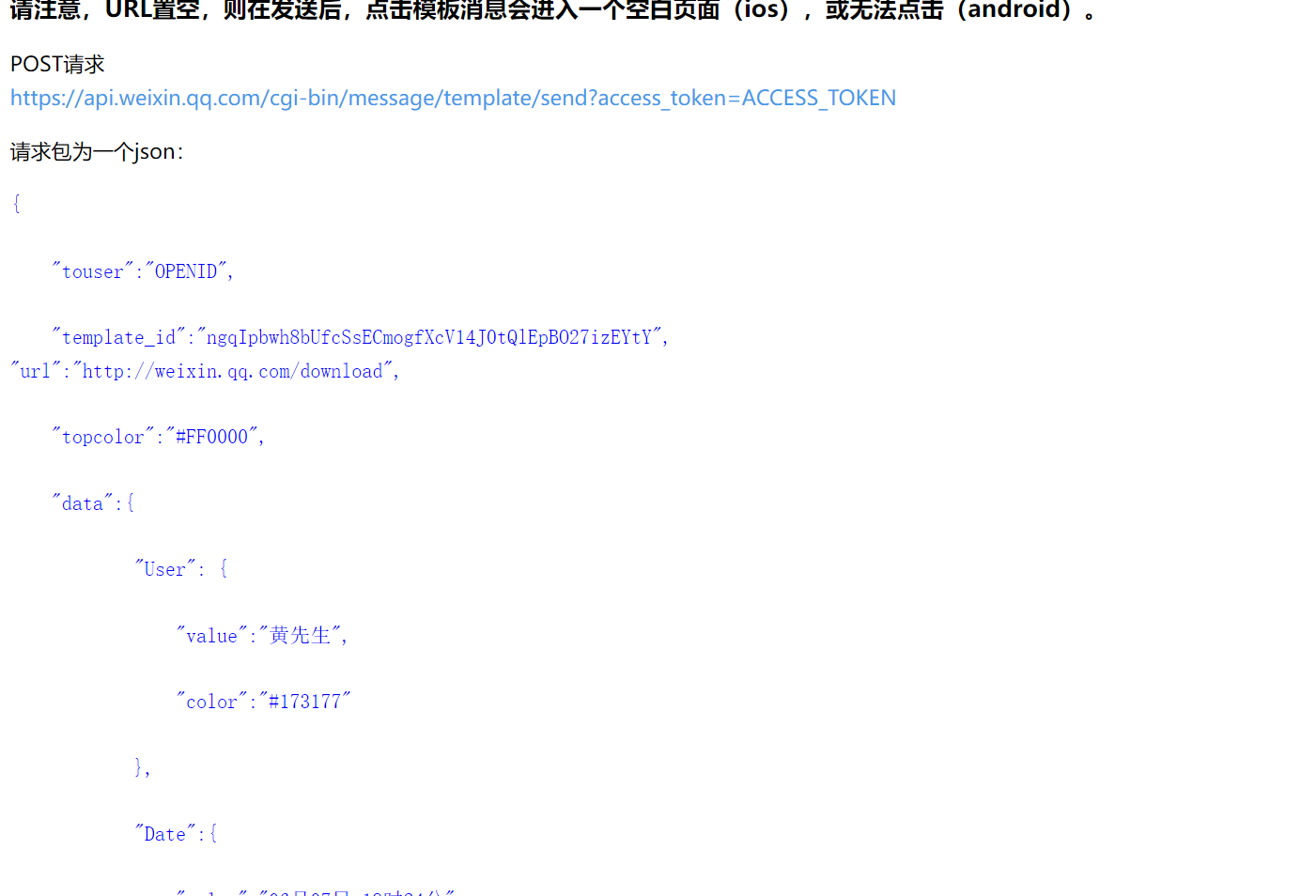
我们只需要按照这种格式发送请求给微信即可。
Java代码
1. 消息实体类
package icu.flycode.sdk.domain.models;
import java.util.HashMap;
import java.util.Map;
public class Message {
// 发送给指定用户
private String touser = "xxx";
// 模板id
private String template_id = "xxx";
// 用户点击可以跳转指定链接
private String url = "xxxx";
private Map<String, Map<String, String>> data = new HashMap<>();
public void put(String key, String value) {
data.put(key, new HashMap<String, String>() {
private static final long serialVersionUID = 7092338402387318563L;
{
put("value", value);
}
});
}
public String getTouser() {
return touser;
}
public void setTouser(String touser) {
this.touser = touser;
}
public String getTemplate_id() {
return template_id;
}
public void setTemplate_id(String template_id) {
this.template_id = template_id;
}
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
public Map<String, Map<String, String>> getData() {
return data;
}
public void setData(Map<String, Map<String, String>> data) {
this.data = data;
}
}
2. 生成Token
package icu.flycode.sdk.utils;
import com.alibaba.fastjson2.JSON;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class WXAccessTokenUtils {
private static final String APPID = "xxx";
private static final String SECRET = "xxxx";
private static final String GRANT_TYPE = "client_credential";
private static final String URL_TEMPLATE = "https://api.weixin.qq.com/cgi-bin/token?grant_type=%s&appid=%s&secret=%s";
public static String getAccessToken() {
try {
String urlString = String.format(URL_TEMPLATE, GRANT_TYPE, APPID, SECRET);
URL url = new URL(urlString);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
int responseCode = connection.getResponseCode();
System.out.println("Response Code: " + responseCode);
if (responseCode == HttpURLConnection.HTTP_OK) {
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String inputLine;
StringBuilder response = new StringBuilder();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
// Print the response
System.out.println("Response: " + response.toString());
Token token = JSON.parseObject(response.toString(), Token.class);
return token.getAccess_token();
} else {
System.out.println("GET request failed");
return null;
}
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
public static class Token {
private String access_token;
private Integer expires_in;
public String getAccess_token() {
return access_token;
}
public void setAccess_token(String access_token) {
this.access_token = access_token;
}
public Integer getExpires_in() {
return expires_in;
}
public void setExpires_in(Integer expires_in) {
this.expires_in = expires_in;
}
}
}
3. 测试调用
@Test
public void test_wx() {
String accessToken = WXAccessTokenUtils.getAccessToken();
System.out.println("accessToken:" + accessToken);
Message message = new Message();
message.put("project", "测试项目");
message.put("review", "测试内容");
String url = String.format("https://api.weixin.qq.com/cgi-bin/message/template/send?access_token=%s", accessToken);
sendPostRequest(url, JSON.toJSONString(message));
}
private static void sendPostRequest(String urlString, String jsonBody) {
try {
URL url = new URL(urlString);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("POST");
conn.setRequestProperty("Content-Type", "application/json; utf-8");
conn.setRequestProperty("Accept", "application/json");
conn.setDoOutput(true);
try (OutputStream os = conn.getOutputStream()) {
byte[] input = jsonBody.getBytes(StandardCharsets.UTF_8);
os.write(input, 0, input.length);
}
try (Scanner scanner = new Scanner(conn.getInputStream(), StandardCharsets.UTF_8.name())) {
String response = scanner.useDelimiter("\\A").next();
System.out.println(response);
}
} catch (Exception e) {
e.printStackTrace();
}
}
4. 测试结果
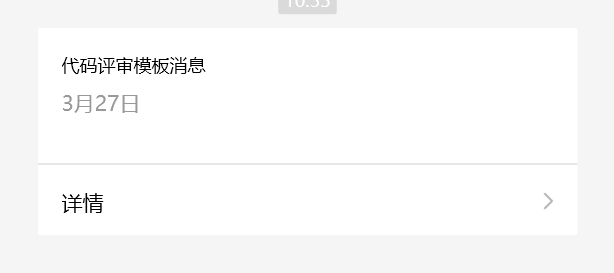
贡献者
flycodeu
版权所有
版权归属:flycodeu